In the ever-evolving world of app development, choosing the right NoSQL database can significantly affect your application’s scalability, performance, and development speed. Two popular contenders in the NoSQL space are MongoDB and Firebase. While both offer flexible, schema-less data storage, they serve different use cases, architectures, and development philosophies.
In this post, we’ll break down the key differences between MongoDB and Firebase to help you decide which is best for your next project.
What is NoSQL, Again?
NoSQL (Not Only SQL) databases are designed for handling unstructured or semi-structured data. Unlike traditional relational databases, they don’t rely on fixed schemas and are optimized for high availability, horizontal scaling, and flexible data models.
Both MongoDB and Firebase fall into the NoSQL category—but with different approaches.
Data Models
MongoDB:
- Document-oriented database.
- Stores data as BSON (Binary JSON) documents inside collections.
- Ideal for nested, complex data structures.
- Similar to JSON, which makes it intuitive for developers coming from web backgrounds.
Example:
{ "name": "Alice", "email": "[email protected]", "orders": [ { "id": 1, "item": "Laptop" }, { "id": 2, "item": "Mouse" } ] }
Firebase (Firestore):
- Also document-based, but follows a slightly different hierarchy.
- Data is stored as documents inside collections, and documents can also have subcollections (but not deeply nested objects).
- Designed for flat and scalable data—nested writes and queries can be more restricted than in MongoDB.
Example:
/users/{userId} /users/{userId}/orders/{orderId}
Real-Time Capabilities
MongoDB:
- Real-time support with MongoDB Change Streams and tools like MongoDB Realm or via additional setup (e.g., using Socket.io).
- Not real-time by default—requires extra configuration.
Firebase:
- Real-time by design.
- Firebase Realtime Database and Firestore both support out-of-the-box real-time sync.
- Ideal for chat apps, collaborative tools, or live dashboards.
Performance & Scalability
MongoDB:
- Designed for high-throughput operations and horizontal scalability using sharding.
- Can be deployed on-premise or in the cloud (MongoDB Atlas).
- Performance tuning requires more hands-on management.
Firebase:
- Auto-scales with minimal configuration.
- Optimized for mobile and web apps with variable traffic.
- Better suited for apps with lighter workloads or real-time demands.
Security & Access Rules
MongoDB:
- Role-Based Access Control (RBAC).
- You define your own security model, which gives flexibility but requires setup.
Firebase:
- Uses Firestore Security Rules or Realtime Database Rules.
- Declarative and granular—but can become complex in large apps.
- Seamlessly integrates with Firebase Authentication.
Development Experience
MongoDB:
- Flexible and powerful querying (aggregation framework, indexes, etc.).
- Integrates well with backend frameworks like Express.js (via Mongoose).
- More control, especially for server-side applications.
Firebase:
- Developer-friendly SDKs for Android, iOS, and Web.
- Great for frontend-heavy or mobile-first applications.
- Backend-as-a-Service (BaaS) model: many built-in tools like authentication, hosting, analytics.
Pricing
MongoDB:
- Free Community version available.
- MongoDB Atlas has a generous free tier; pricing scales based on storage, throughput, and clusters.
Firebase:
- Generous free tier with daily limits.
- Pricing can grow rapidly for apps with lots of reads/writes or real-time listeners.
When to Use MongoDB
- You need flexible queries and complex aggregations.
- Your app is server-heavy (Node.js, Python, etc.).
- You want control over backend architecture.
- You’re building analytics tools, reporting engines, or microservices.
When to Use Firebase
- You’re building a mobile-first or web app with real-time features.
- You want rapid development with minimal backend setup.
- You need tight integration with Google Cloud and Firebase services.
- Ideal for MVPs, chat apps, and small to medium apps.
Briefly
Both MongoDB and Firebase are powerful NoSQL solutions—but they shine in different areas. MongoDB gives you more control, power, and flexibility on the backend, while Firebase is great for real-time, client-heavy apps with fast development needs.
Ultimately, the best choice depends on your project’s requirements, your team’s expertise, and how much backend you want to manage.
What has your experience been with MongoDB or Firebase? Let me know in the comments!
Would you like me to help you tailor this for SEO, add a title image, or post formatting (Markdown, HTML, etc.)?
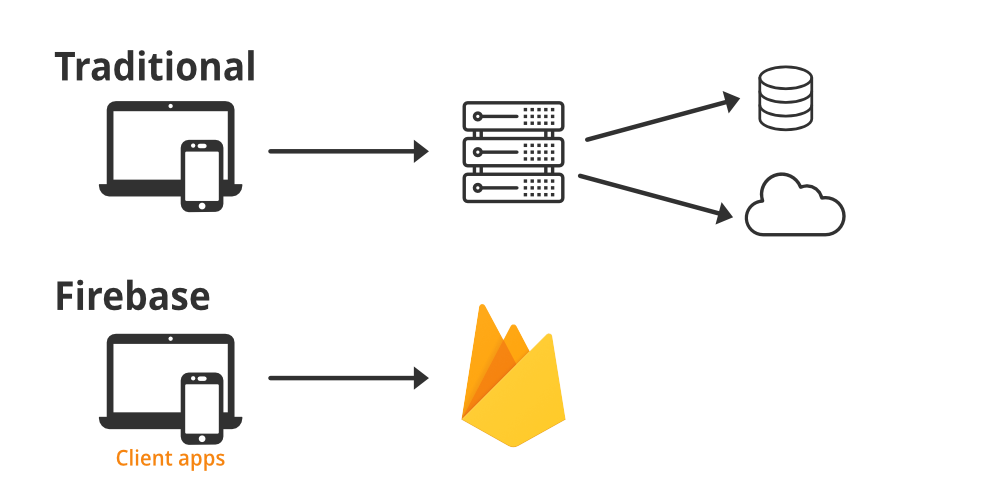
Example Scenario
Let’s say we’re building a shopping app, and we want to store data for a user who has placed a couple of orders.
MongoDB Example
In MongoDB, we can embed related data directly inside a document. This is often called denormalization, and it works well when data is typically accessed together.
{ "_id": "user_123", "name": "Alice", "email": "[email protected]", "orders": [ { "order_id": "order_001", "date": "2025-05-15", "items": [ { "name": "Laptop", "price": 1200 }, { "name": "Mouse", "price": 25 } ] }, { "order_id": "order_002", "date": "2025-05-14", "items": [ { "name": "Keyboard", "price": 75 } ] } ] }
✅ Pros: All data is in one place — easy to query and read.
⚠️ Cons: If the orders become too large, the document can become bloated.
Firebase Firestore Example
In Firestore, the best practice is to normalize your data by separating it into collections and subcollections.
/Users/user_123 { "name": "Alice", "email": "[email protected]" } /Users/user_123/Orders/order_001 { "date": "2025-05-15", "items": [ { "name": "Laptop", "price": 1200 }, { "name": "Mouse", "price": 25 } ] } /Users/user_123/Orders/order_002 { "date": "2025-05-14", "items": [ { "name": "Keyboard", "price": 75 } ] }
✅ Pros: Each order is a separate document—ideal for real-time updates and scaling.
⚠️ Cons: Requires multiple reads to fetch user data + all orders.
Summary of Key Differences
Feature | MongoDB | Firebase Firestore |
---|---|---|
Data modeling style | Embedded documents (denormalized) | Subcollections (normalized) |
Ideal for | Complex queries, backend apps | Real-time sync, mobile/web apps |
Querying | Powerful aggregation + filters | Limited joins, but real-time querying |
Scalability | Manual tuning (sharding) | Auto-scaling with limits |