Introduction to MongoDB and Its NoSQL Work
What is MongoDB?
MongoDB is a popular, open-source NoSQL database designed to store and manage large volumes of unstructured or semi-structured data. Unlike traditional relational databases (RDBMS) that use tables and rows, MongoDB uses a flexible document-oriented model.
Key Features of MongoDB:
- Document-Based Storage: Data is stored in BSON (Binary JSON) format, allowing for nested fields and varying structures within a collection.
- Schema-less Design: Collections do not enforce a strict schema, making it easier to modify the structure of stored documents.
- Horizontal Scalability: Supports sharding, allowing it to distribute data across multiple servers.
- High Availability: Provides replica sets for automatic failover and data redundancy.
- Rich Query Language: Offers powerful querying capabilities including filtering, aggregation, and indexing.
MongoDB in the NoSQL Landscape
MongoDB belongs to the NoSQL family of databases, a term that stands for “Not Only SQL“. NoSQL databases were developed to overcome the limitations of relational databases, especially in the era of big data, real-time applications, and cloud computing.
There are four main types of NoSQL databases:
- Document Stores (e.g., MongoDB)
- Key-Value Stores (e.g., Redis, DynamoDB)
- Column-Family Stores (e.g., Cassandra, HBase)
- Graph Databases (e.g., Neo4j)
Use Cases of MongoDB:
- Content management systems
- Real-time analytics platforms
- Mobile applications
- Internet of Things (IoT) systems
- Catalogs and inventory management
Advantages of MongoDB in NoSQL Work:
- Greater flexibility in handling data with varying structures
- Easier to scale horizontally
- Ideal for agile development due to its dynamic schema
- Efficient storage and retrieval of complex data types like arrays and nested objects
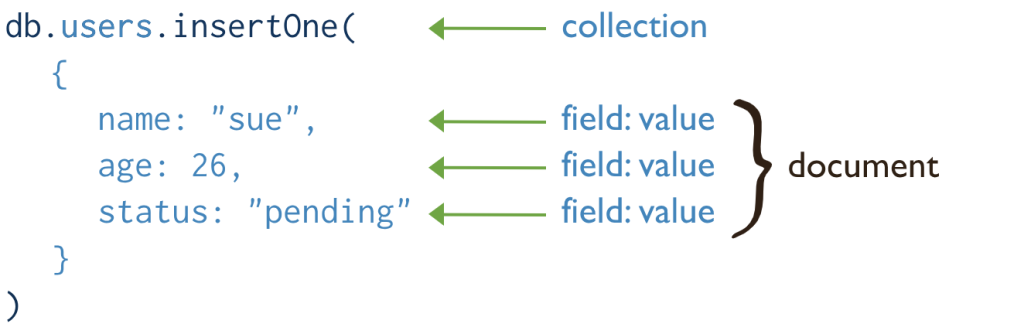
Using Statements in MongoDB vs SQL
MongoDB Does Not Use SQL
MongoDB uses its own query language, which is object-based and typically written in JSON-like syntax. You interact with MongoDB using methods like .find()
, .insertOne()
, .updateOne()
, etc.
But for easier understanding, here’s a comparison of SQL vs MongoDB operations:
1. SELECT Statement
SQL | MongoDB |
---|---|
SELECT * FROM users | db.users.find() |
SELECT name FROM users | db.users.find({}, { name: 1, _id: 0 }) |
SELECT * FROM users WHERE age > 25 | db.users.find({ age: { $gt: 25 } }) |
2. INSERT Statement
SQL | MongoDB |
---|---|
INSERT INTO users (name, age) VALUES ('John', 30) | db.users.insertOne({ name: "John", age: 30 }) |
3. UPDATE Statement
SQL | MongoDB |
---|---|
UPDATE users SET age = 31 WHERE name = 'John' | db.users.updateOne({ name: "John" }, { $set: { age: 31 } }) |
4. DELETE Statement
SQL | MongoDB |
---|---|
DELETE FROM users WHERE age < 20 | db.users.deleteMany({ age: { $lt: 20 } }) |
📊 5. Aggregation (GROUP BY)
SQL | MongoDB |
---|---|
SELECT age, COUNT(*) FROM users GROUP BY age | db.users.aggregate([ { $group: { _id: "$age", count: { $sum: 1 } } } ]) |
MongoDB Query Basics
MongoDB queries use this pattern:
db.collection.method(query, projection)
collection
: the table-like structure (e.g.,users
)query
: filters documents (like WHERE in SQL)projection
: selects which fields to return (like SELECT columns)
Tools to Help
MongoDB provides:
- MongoDB Shell: Command-line interface
- MongoDB Compass: GUI for visualizing databases
- Drivers: For using MongoDB with Python, Node.js, Java, etc.
Great question! Understanding the relationship between tables in SQL and documents in MongoDB is key to working effectively with both. Here’s a comparison:
Tables in SQL vs Documents in MongoDB
Concept | SQL (Relational DB) | MongoDB (NoSQL Document DB) |
---|---|---|
Database | Database | Database |
Table | Table | Collection |
Row | Row (Record) | Document (BSON/JSON) |
Column | Column (Field) | Field (Key-value pair) |
Primary Key | Unique identifier (e.g., id ) | _id field (automatically generated) |
Relationships | Foreign keys, Joins | Embedded documents or references |
Relationships in SQL vs MongoDB
1. One-to-One
SQL (Relational) | MongoDB (NoSQL) |
---|---|
Use foreign key to link tables | Embed one document inside another |
SQL Example:
CREATE TABLE users (id INT, name TEXT); CREATE TABLE profiles (user_id INT, bio TEXT, FOREIGN KEY(user_id) REFERENCES users(id));
MongoDB Example:
{ _id: ObjectId("..."), name: "Alice", profile: { bio: "Software engineer" } }
2. One-to-Many
SQL (Relational) | MongoDB (NoSQL) |
---|---|
Parent table links to many rows in child table | Embed array of documents or use references |
Embedded Example in MongoDB:
{ name: "Alice", orders: [ { item: "Book", price: 12 }, { item: "Pen", price: 2 } ] }
Referenced Example in MongoDB:
// users collection { _id: 1, name: "Alice" } // orders collection { user_id: 1, item: "Book", price: 12 }
3. Many-to-Many
SQL (Relational) | MongoDB (NoSQL) |
---|---|
Use junction (join) tables | Use arrays of references or multiple collections |
SQL Example:
CREATE TABLE students (id INT, name TEXT); CREATE TABLE courses (id INT, title TEXT); CREATE TABLE enrollment (student_id INT, course_id INT);
MongoDB Example with References:
// students { _id: 1, name: "Bob", courses: [101, 102] } // courses { _id: 101, title: "Math" } { _id: 102, title: "Science" }
🧠 Best Practices
- Use embedding for closely related data (e.g., user and profile).
- Use referencing for loosely related or large datasets (e.g., blog and comments).
- MongoDB does not support joins natively like SQL, but the aggregation framework and
$lookup
can simulate joins.